In this tutorial I will show you how to blur an image in python.
To blur an Image:
You can use OpenCV LIbrary, which provides various image processing methods including blurring.
Install the opencv-python Library:
pip install opencv-python
Example Program:
import cv2 try: image = cv2.imread('angelina.jpg') blurred_image = cv2.GaussianBlur(image, (15, 15), 0) cv2.imshow('Original Image', image) cv2.imshow('Blurred Image', blurred_image) cv2.waitKey(0) cv2.destroyAllWindows() output_path = 'blurred_image.jpg' cv2.imwrite(output_path, blurred_image) cv2.waitKey(0) cv2.destroyAllWindows() except Exception as e: print(f"An error occurred: {e}")
OUTPUT:
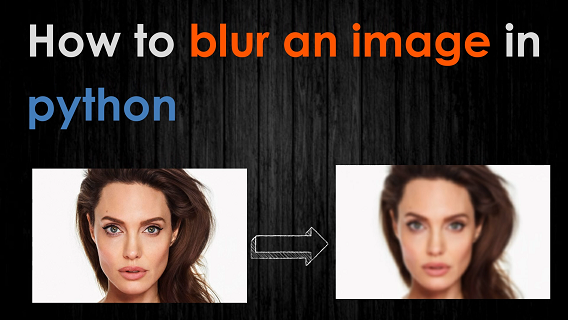
VIDEO GUIDE:
Post your comments / questions
Recent Article
- FieldError: Cannot resolve keyword 'id' into field in Django project
- How to hide the ID field from the Django admin?
- It is impossible to add a non nullable field without specifying a default. Django error
- ImportError: cannot import name 'url' from 'django.conf.urls' - Django Error
- How to Enable Virtualization in BIOS Security Settings in Intel Processors For Android Studio?
- Dependency 'androidx.activity:activity:1.8.0' requires libraries and applications that depend on it.
- AttributeError: 'NoneType' object has no attribute 'get_text' - Python
- ModuleNotFoundError: No module named 'openpyxl' - Python
Related Article