In this tutorial I will show you how to bind data from json file in angular. I created a json file named data.json in local asset folder. Also imported data.json file in appcomponent and created an interface named "post" and declare properties and then assigned postjson to the interface object.If you got this following error "cannot find module consider using '--resolvejsonmodule' to import module with json extension angular" then click 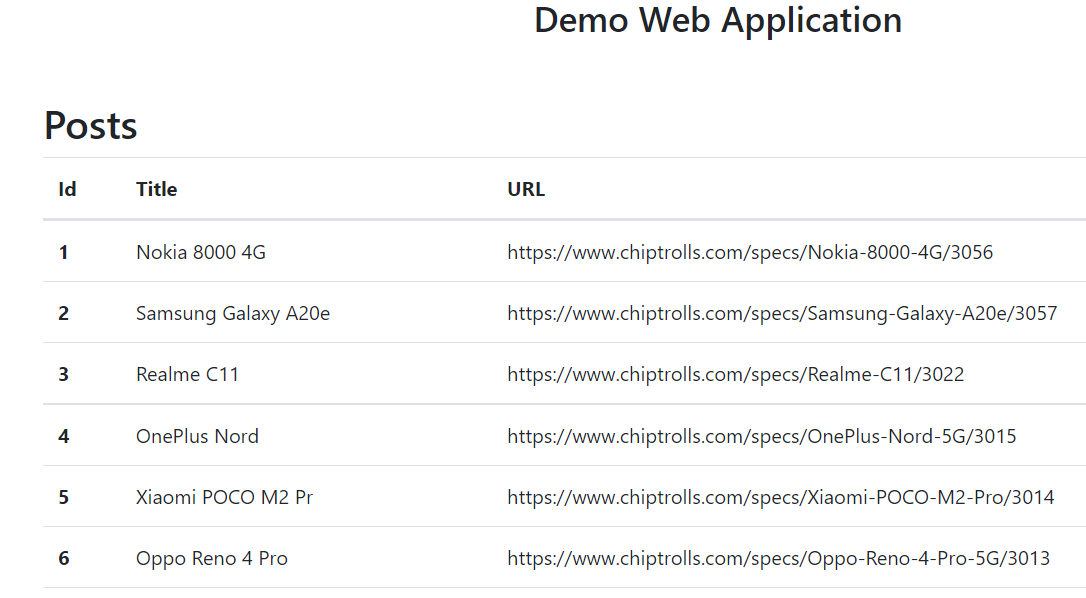
data.json file:
[
{
"id": 1,
"title": "Nokia 8000 4G",
"url": "https://www.chiptrolls.com/specs/Nokia-8000-4G/3056"
},
{
"id": 2,
"title": "Samsung Galaxy A20e",
"url": "https://www.chiptrolls.com/specs/Samsung-Galaxy-A20e/3057"
},
{
"id": 3,
"title": "Realme C11",
"url": "https://www.chiptrolls.com/specs/Realme-C11/3022"
},
{
"id": 4,
"title": "OnePlus Nord",
"url": "https://www.chiptrolls.com/specs/OnePlus-Nord-5G/3015"
},
{
"id": 5,
"title": "Xiaomi POCO M2 Pr",
"url": "https://www.chiptrolls.com/specs/Xiaomi-POCO-M2-Pro/3014"
},
{
"id": 6,
"title": "Oppo Reno 4 Pro",
"url": "https://www.chiptrolls.com/specs/Oppo-Reno-4-Pro-5G/3013"
},
{
"id": 7,
"title": "Huawei Enjoy 20 Pro",
"url": "https://www.chiptrolls.com/specs/Huawei-Enjoy-20-Pro/3012"
},
{
"id": 8,
"title": "Vivo X50 Pro",
"url": "https://www.chiptrolls.com/specs/Vivo-X50-Pro/3011"
},
{
"id": 9,
"title": "LG Q61",
"url": "https://www.chiptrolls.com/specs/LG-Q61/3008"
},
{
"id": 10,
"title": "Apple iPhone 12 Pro Max",
"url": "https://www.chiptrolls.com/specs/Apple-iPhone-12-Pro-Max/3006"
}]
blog.component.ts:
import { Component, OnInit } from '@angular/core';
import postJson from 'src/assets/data.json';interface post {id: number;title: string;url: string;}@Component({selector: 'app-blog',templateUrl: './blog.component.html',styleUrls: ['./blog.component.css']})export class BlogComponent implements OnInit {posts: post[] = postJson;constructor() { }ngOnInit(): void {}}
blog.component.html:
<div class="container mt-5">
<h2>Posts</h2>
<table class="table">
<thead>
<tr>
<th>Id</th>
<th>Title</th>
<th>URL</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let item of posts">
<th scope="row">{{ item.id }}</th>
<td>{{ item.title }}</td>
<td>{{ item.url }}</td>
</tr>
</tbody>
</table>
</div>
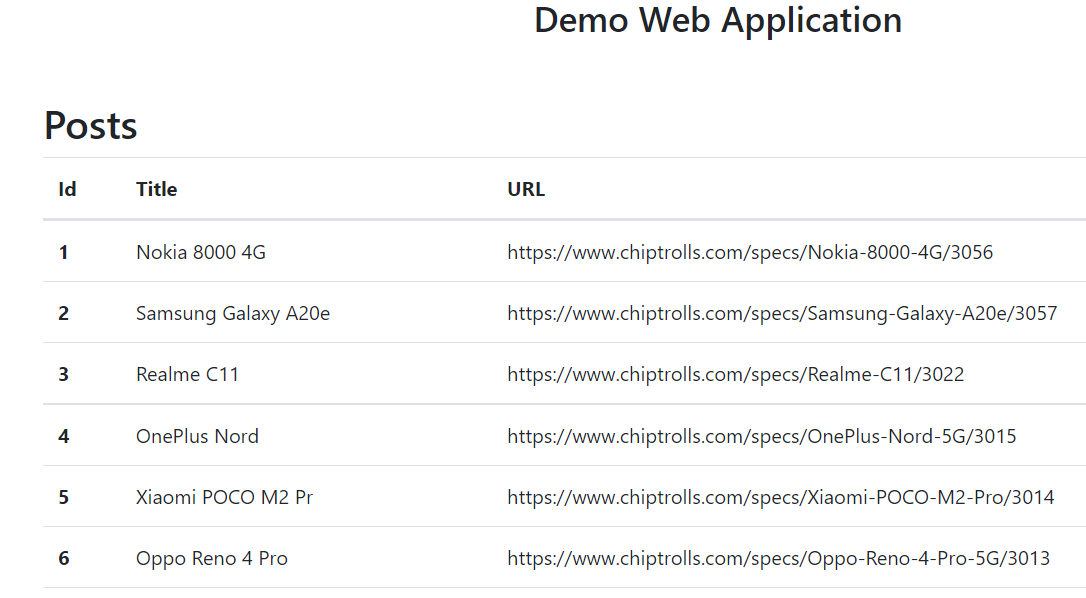
Post your comments / questions
Recent Article
- How to restrict access to the page Access only for logged user in Django
- Migration admin.0001_initial is applied before its dependency admin.0001_initial on database default
- Add or change a related_name argument to the definition for 'auth.User.groups' or 'DriverUser.groups'. -Django ERROR
- Addition of two numbers in django python
- The request was aborted: Could not create SSL/TLS secure channel -Error in Asp.net
- FieldError: Cannot resolve keyword 'id' into field in Django project
- How to hide the ID field from the Django admin?
- It is impossible to add a non nullable field without specifying a default. Django error
Related Article