In this tutorial I will show you how to convert csv to json in python.
To convert csv to json:
we have to import csv and json packages. Use csv.DictReader() to read the lines of the csv file and then add to the array. To convert object to the JSON string using json.dumps() and write to JSON String to json file.
CODE:
import csvimport jsonjsonArr = []with open('shampoo_sales.csv', encoding='utf-8') as csvfile:csvReader = csv.DictReader(csvfile)for row in csvReader:jsonArr.append(row)with open('shampoo_sales.json', 'w', encoding='utf-8') as jsonfile:jsonString = json.dumps(jsonArr, indent=4)jsonfile.write(jsonString)
OUTPUT:
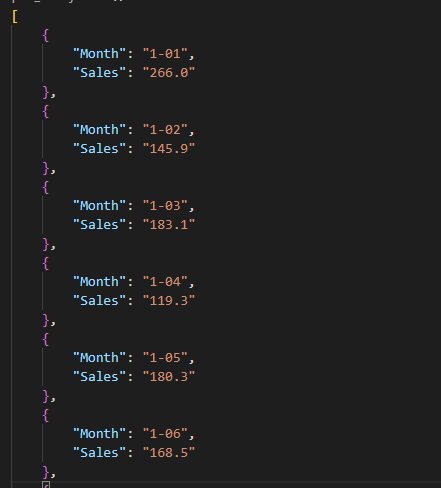
Post your comments / questions
Recent Article
- How to restrict access to the page Access only for logged user in Django
- Migration admin.0001_initial is applied before its dependency admin.0001_initial on database default
- Add or change a related_name argument to the definition for 'auth.User.groups' or 'DriverUser.groups'. -Django ERROR
- Addition of two numbers in django python
- The request was aborted: Could not create SSL/TLS secure channel -Error in Asp.net
- FieldError: Cannot resolve keyword 'id' into field in Django project
- How to hide the ID field from the Django admin?
- It is impossible to add a non nullable field without specifying a default. Django error
Related Article